Console Input and Output
Overview
In this lesson, students will learn how to accept input from a user and produce console output.
Learning Objectives
- 1.1.C.4 Write and interpret output statements.
- 1.1.C.5 Write and interpret input statements for values being provided from an external source.
Skills
- S1.B Design algorithms for a program.
- S1.D Explain the impact design has on data storage.
- S2.A Write program code and implement algorithms.
- S2.C Analyze an algorithm and program code for correctness.
Student Outcomes
Students will be able to:
- prompt users for input
- accept input into the program from a console
- write output statements to the console
Duration: 1 class period
Resources
Warm-up / Motivate
Have students look at their favorite app on their phone. If allowed, have them use it for a few minutes or have them think about what it takes to use it.
- How do the interact with the app?
- What data do they enter?
- Is there data that doesn't use the keyboard, like pressing a button or drawing on the screen of their phones?
- What data is produced by the program? What is the output?
Learn
Either as a group or on their own, have students review tutorial: Learn: Introduction to Console Input and Output
Debrief: Take time to answer any questions that students might have. Review their answers to the guiding questions provided.
Practice
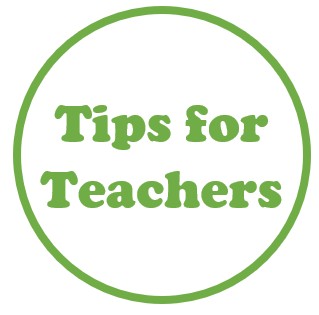
**Pair Programming** is a technique that students can use to collaboratively write a program. With pair programming, students take one of two roles: driver or programmer. The driver does not touch the keyboard, but tells the programmer what to write. The programmer is at the keyboard, writing the code provided by the driver. If the programmer disagrees with the driver they are to discuss and come up with a solution. After a specified amount of time (10 - 20 minutes), students switch roles.
Have students complete the practice using the Pair Programming technique: Practice: Reading and Writing Console Input and Output.
Apply
Have students complete the mini-lab exercises: Mini-Labs: Input and Output to the Console
Wrap-up / Extension
Have students write a short program where they take in the number of hours worked and the program tells them their pay if they get paid $8 an hour. Ask students to do some planning by answering these questions:
- What data is the program using?
- number of hours worked
- amount they get paid per hour
- What data is being produced?
- the amount they would get paid
- What is the type of this data?
- hours worked is an
int
or a double
if they want to allow partial hours worked
- amount they get paid is a constant and in this case can be stored as an
int
- amount paid can be an
int
if the other data values are both int
, otherwise the type double
should be used
- What are good variables names for this data? Some examples:
- hoursWorked
- hourlyRate
- amountPaid
- What are the steps to write the program?
- declare variables and constants
- prompt the user to input the hours worked
- compute the amount they would get paid
- output this value to the user
Once they have a plan, they should put the code into the IDE and run it.
Extension
Have students modify the program to obtain the pay rate from the user as well.
Next Lesson
Lesson Plan: Formatting Output and Text Blocks