What is the Ternary Conditional Operator?
The Ternary Conditional Operator results in a value based on whether a Boolean expression is evaluated to true or false.
The ternary conditional operator consists of a question mark (?) and a colon (:), as follows:
<Boolean expression> ? <expression if true> : <expression if false>
Ternary Conditional Operator consists of three expressions:
<Boolean expression> ? <expression 1> : <expression 2>
The first expression is a Boolean expression
expression 1 and expression 2 can be any expression:
- A value
- A non-void method call
- Another ternary conditional operator expression
Example 1: Even or Odd
To determine whether x
is even or odd, we can use the mod operator to determine whether x
is divisible by 2. If the result of x % 2
is 0, then x
is even otherwise, it is odd.
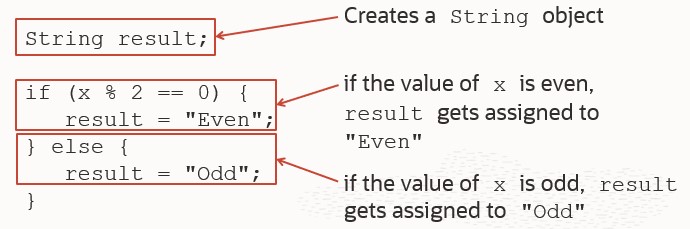
To re-write this if statement using ternary conditional operators, we will put the boolean expression in first.
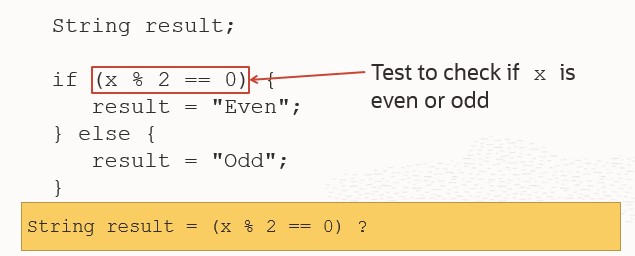
The ?
operator is used after the boolean expression. Think of it as asking if the boolean expression is true. If it is true, then the next part of the expression is evaluated and Even is assigned to result.
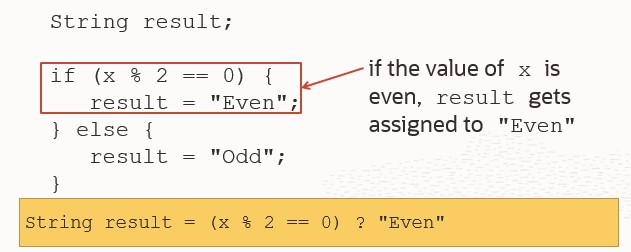
A colon (:
) is used to separate what happens when the boolean expression is true and when it evaluates to false. If the boolean expression evaluates to false in this example, Odd is assigned to result.
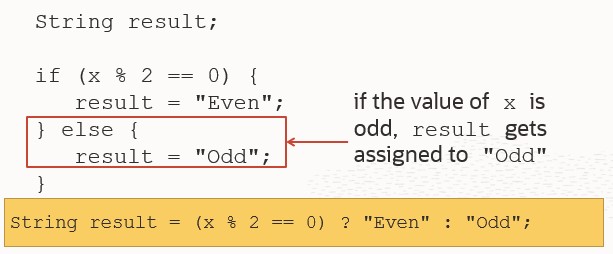
To Recap
These two statements are equivalent just written differently.
Produces the same result as:
We could use the ternary conditional operator to print whether the value assigned to x
is even or odd simply by putting the expression inside a System.out.println
statement:
Example 2: Calling Methods
Consider a class called Calculator that has methods to calculate the sum and positive difference of two values as follows:
public double sum(double value1, double value2);
public double posDifference(double value1, double value2);
Based on a String input of +
for sum and +-
for positive difference, we can call the appropriate method:
Example 3: Nested Ternary
Expanding on the last example.
Assume Calculator
has product and quotient methods as well.
Re-written as an if..else statement:
Ternary and void Method Calls
Remember, when using the Ternary Conditional Operator, the result is a value. You can not use it to call void methods. For example, consider a Robot class that has methods for turning the robot to the left and right as follows:
public void turnRight()
public void turnLeft()
Assuming randy is a properly declared Robot and direction is an int variable, we can NOT write any of the following:
(direction == 39) ? randy.turnRight() : randy.turnLeft();
boolean b = (direction == 39) ? randy.turnRight() : randy.turnLeft();
System.out.println ((direction == 39)
? randy.turnRight() : randy.turnLeft());
Instructional Ideas to Engaging Students
- Have students modify if statements they have written in other projects to use the Ternary Conditional Operator instead.
- Create your own parsons type problems. Put a code description along with the expressions needed to write the code into a zip-lock bag and have students assemble as part of a class warm-up.
- Provide students with prompts and have them write both the if and ternary solutions side-by-side.
Ternary Conditional Operators and the AP CSA Free Response Questions
Things to Note on Using Ternary Conditional Operator and the AP CSA FRQs
- Ternary Conditional Operators are OUTSIDE the #APCSA Java Subset.
- Students can write correct solutions using the Ternary Conditional Operator
- This operator can be used in any FRQ, but may be seen more often in Question 1.
Example 1: 2024 Free Response Question 1
In 2024, question 1 can be re-written using the ternary conditional operator. The full question and solution can be found here.
To summarize, this question has a class called Feeder that has an instance variable currentFood and two methods simulateOneDay and simulateManyDays. Students are asked to implement these two methods. We are looking at writing the simulateOneDay method.
To simulate a day at the bird feeder, students will need to do the following:
- generate a random number to determine if a bear visited the bird feeder.
- If a bear came to the feeder, the value of currentFood would be set to 0.
- If there is no bear, then another number is simulate the amount of food each bird will consume.
- If the amount of food the birds consumed exceeds the amount in the feeder, the value of currentFood is 0, otherwise the amount consumed is subtracted from currentFood.
An example solution is as follows. Run it several times to see the results:
This following part of the if statement can be re-written using the ternary conditional operator.
The original if statement:
if (birdConsumed >= currentFood) {
currentFood = 0;
}
else {
currentFood = currentFood - birdConsumed;
}
Re-written as:
currentFood = (birdConsumed >= currentFood) ? 0 : (currentFood – birdConsumed);
This ternary conditional operator expression is nested inside another if statement as follows:
if (bearPresent) {
currentFood = 0;
}
else {
currentFood = (birdConsumed >= currentFood) ? 0 : (currentFood – birdConsumed);
}
This can be re-written using nested ternary conditional operators as following. Run it several times to see the results:
Scoring and Teaching
- Student solutions that use correct Java syntax can earn full credit for their solution
- Questions are written with an in AP Java Subset solution in mind
- Students should be encouraged to write solutions within the AP Java Subset
Resources
Calculator - Using Conditionals and Lambda Mini-Lab